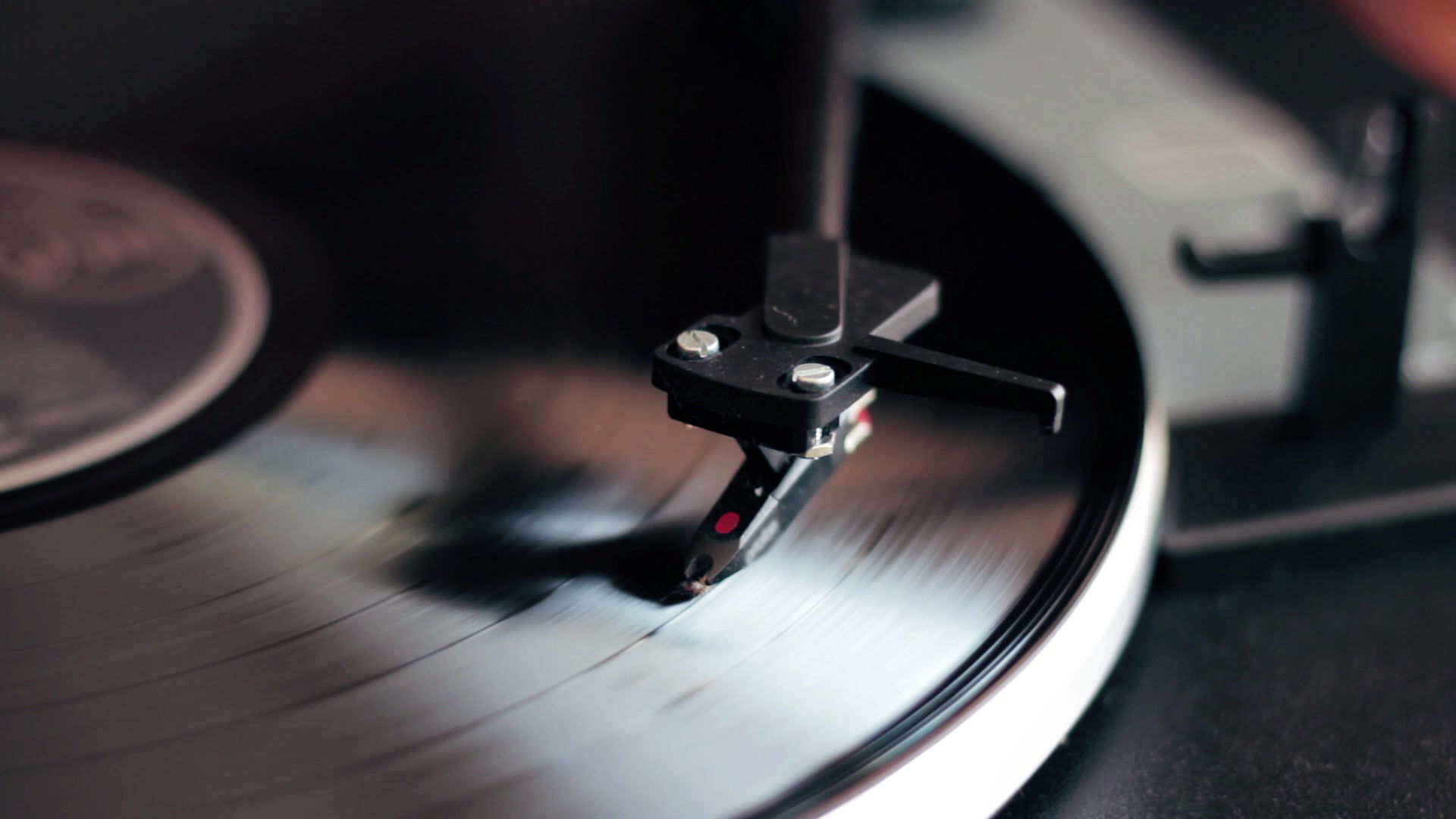
HLessJon Tutorials
Change LinearLayout from horizontal to vertical orientation
Defining the size of layout elements using layout_width and layout_height:
android:layout_width="100dp"
android:layout_height="60dp"
Use relative width and height for layout elements: wrap_content and match_parent
Use of layout_weight:
android:layout_height="0dp"
android:layout_weight="1"
Create a gap between elements and the edge of the screen:
android:layout_marginRight="20dp"
android:layout_marginTop="15dp"
Paddings is very similiar to margins:
android:paddingLeft="20dp"
Changing font size, style, typeface and color:
android:textSize="15dp"
android:textStyle="bold"
android:typeface="serif"
android:textColor="#0000CD
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.mycompany.xmlexample.MainActivity">
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="200dp"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:id="@+id/linearLayout">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Linear1"
android:id="@+id/textView1"
android:background="#ff0000" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Linear2"
android:id="@+id/textView2"
android:background="#ffd900" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Linear3"
android:id="@+id/textView3"
android:background="#009dff" />
</LinearLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="200dp"
android:layout_below="@+id/linearLayout"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Relative1"
android:id="@+id/RtextView1"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:background="#ff0000" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Relative"
android:id="@+id/RtextView2"
android:layout_alignBottom="@+id/RtextView1"
android:layout_toRightOf="@+id/RtextView1"
android:layout_toEndOf="@+id/RtextView1"
android:background="#ffff00" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Relative3"
android:id="@+id/RtextView3"
android:layout_alignBottom="@+id/RtextView2"
android:layout_toRightOf="@+id/RtextView2"
android:layout_toEndOf="@+id/RtextView2"
android:background="#0093a6" />
</RelativeLayout>
</RelativeLayout>