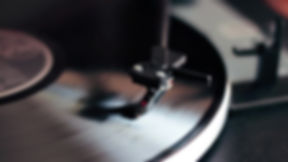
HLessJon Tutorials
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ff0000"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceLarge"
android:text="Hello Dave"
android:id="@+id/textView2"
android:layout_gravity="center_horizontal"
android:layout_marginTop="30dp" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="New Button"
android:id="@+id/button2"
android:layout_margin="100dp"
android:layout_gravity="center_horizontal" />
</LinearLayout>
package com.mycompany.myapplicationx;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
//you will need to import throughout the creation of this code.
//The class needs to extend Fragment
public class MyFrag extends Fragment {
//The newInstance() method return the reference to fragment
public static MyFrag newInstance() {
MyFrag fragment = new MyFrag();
return fragment;
}
//MyFragment is the constructor method of our class.
//this is a java thing. Google it as you this is basic
//java that you need to know.
public MyFrag() {
}
//since we have a button and textview on the xml will use these two
//variable to connect to them.
Button button2;
TextView daveText;
//this method links the fragment to the layout
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
//connecting the fragment to our layout, new_frag
View rootView = inflater.inflate(R.layout.new_frag, container, false);
//then the btn and the textview
button2 = (Button) rootView.findViewById(R.id.button2);
daveText = (TextView) rootView.findViewById(R.id.textView2);
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if(daveText.getText().toString().contains("Hello Dave")){
daveText.setText("I'm sorry Dave I can't do that.");
}else daveText.setText("Hello Dave");
}
});
// return our View
return rootView;
}
} // This is the end. But we still have to change the mainactivity.java to work with it.
switch (position) {
case 0:
return PlaceholderFragment.newInstance(position + 1);
case 1:
return MyFrag.newInstance();
// default: return MyFrag.newInstance();
/* You could use default here instead*/
//STILL NEED TO CHANGE THE getCount!!
}
return null; //if you use default, you would not need to return null
}
**************************
//added the new getCount
public int getCount() {
// Show 2 total pages.
return 2;
}