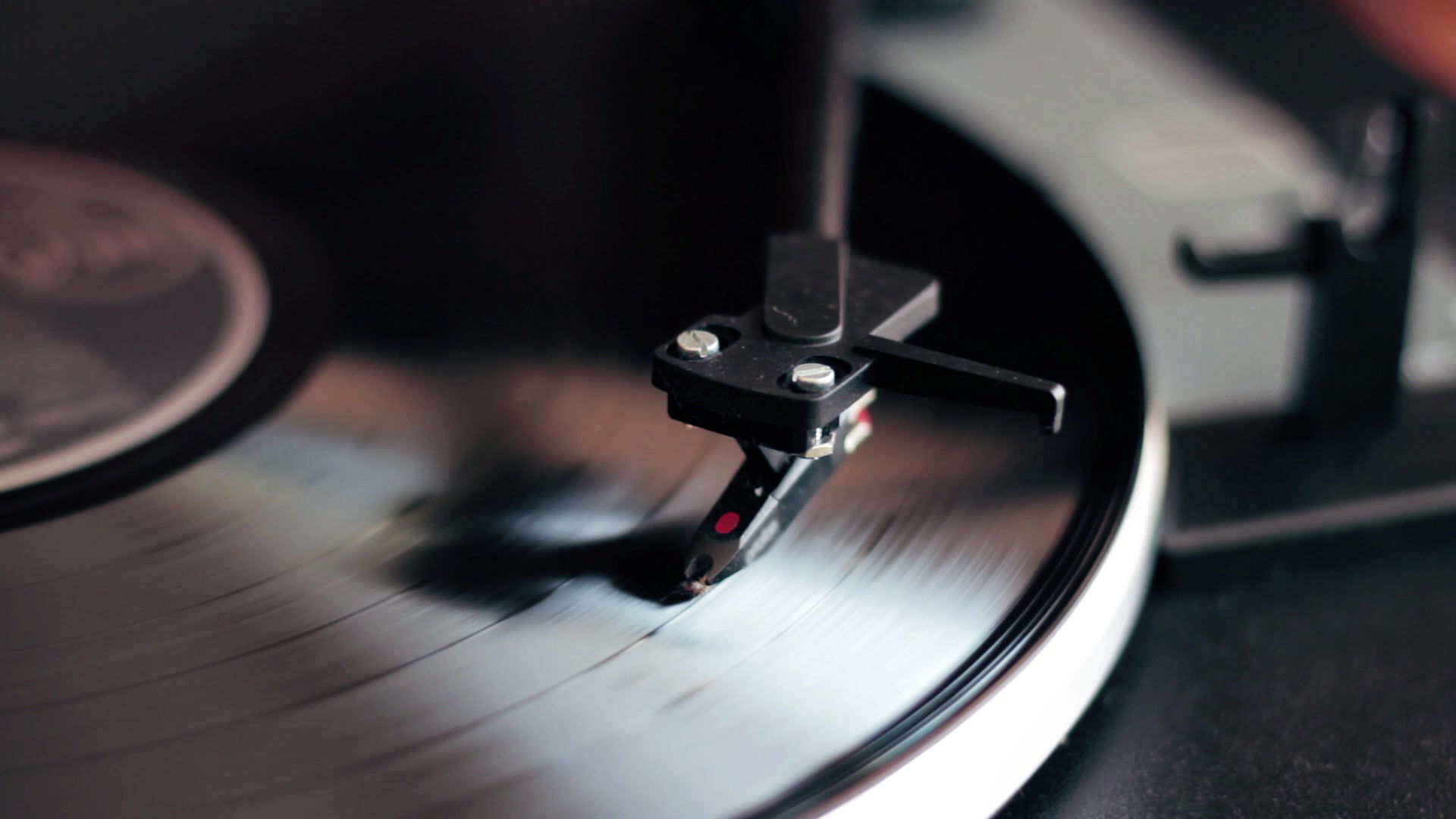
HLessJon Tutorials
Scroll down for the code.
You will need a background image in your drawable folder or you can simply delete the line
android:background="@drawable/bkg"
from activity_main.xml
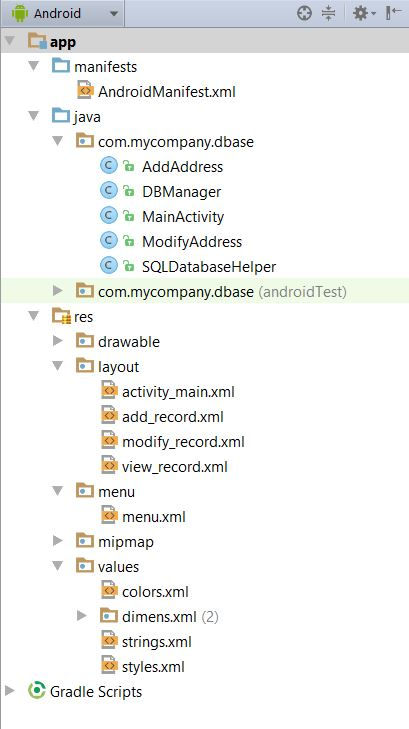
package com.mycompany.dbase;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
public class AddAddress extends Activity implements OnClickListener {
private Button addTodoBtn;
private EditText nameEditText;
private EditText addEditText;
private DBManager dbManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setTitle("Add Record");
setContentView(R.layout.add_record);
nameEditText = (EditText) findViewById(R.id.add_name_edittext);
addEditText = (EditText) findViewById(R.id.add_address_edittext);
addTodoBtn = (Button) findViewById(R.id.add_add_record);
dbManager = new DBManager(this);
dbManager.open();
addTodoBtn.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.add_add_record:
final String name = nameEditText.getText().toString();
final String address = addEditText.getText().toString();
dbManager.insert(name, address);
//After inserting data in database you should close the database connection.
dbManager.close(); // Closing database connection
Intent main = new Intent(AddAddress.this, MainActivity.class)
.setFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
startActivity(main);
break;
}
}
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////
package com.mycompany.dbase;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
public class DBManager {
private SQLDatabaseHelper dbHelper;
private Context context;
private SQLiteDatabase database;
public DBManager(Context charlie) {
context = charlie;
}
public DBManager open() throws SQLException {
dbHelper = new SQLDatabaseHelper(context);//instantiate your subclass
database = dbHelper.getWritableDatabase();//put your DB into write mode.
return this;
}
//this is what will close a dbase.
public void close() {
dbHelper.close();}
//CRUD operations. (Create, Read, Update and Delete)
public void insert(String name, String address) {
ContentValues contentValue = new ContentValues();
contentValue.put(SQLDatabaseHelper.NAME, name);
contentValue.put(SQLDatabaseHelper.ADDRESS, address);
database.insert(SQLDatabaseHelper.TABLE_NAME, null, contentValue);
}
public Cursor fetch() {
String[] columns = new String[] { SQLDatabaseHelper._ID, SQLDatabaseHelper.NAME,
SQLDatabaseHelper.ADDRESS };
Cursor cursor = database.query(SQLDatabaseHelper.TABLE_NAME, columns, null, null, null,
null, null);
if (cursor != null) {
cursor.moveToFirst();
}
return cursor;
}
public int update(long _id, String name, String address) {
ContentValues contentValues = new ContentValues();
contentValues.put(SQLDatabaseHelper.NAME, name);
contentValues.put(SQLDatabaseHelper.ADDRESS, address);
return database.update(SQLDatabaseHelper.TABLE_NAME, contentValues, SQLDatabaseHelper.
_ID + " = " + _id, null);
}
public void delete(long _id) {
database.delete(SQLDatabaseHelper.TABLE_NAME, SQLDatabaseHelper._ID + "=" + _id, null);
}
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////
package com.mycompany.dbase;
import android.content.Intent;
import android.database.Cursor;
import android.os.Bundle;
import android.support.v4.widget.SimpleCursorAdapter;
import android.support.v7.app.AppCompatActivity;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
private String id;
private String name;//title
private String address;
private DBManager dbManager;
private ListView listView;
private SimpleCursorAdapter adapter;
final String[] from = new String[] { SQLDatabaseHelper._ID,
SQLDatabaseHelper.NAME, SQLDatabaseHelper.ADDRESS };
final int[] to = new int[] { R.id.view_id, R.id.view_name, R.id.view_address };
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
dbManager = new DBManager(this);
dbManager.open();
Cursor cursor = dbManager.fetch();
listView = (ListView) findViewById(R.id.list_view);
listView.setEmptyView(findViewById(R.id.empty));
//SimpleCursorAdapter adapter to map columns from a cursor to view defined in the XML
adapter = new SimpleCursorAdapter(this, R.layout.view_record, cursor, from, to, 0);
/* notifyDataSetChanged() notifies the attached observers that
the underlying data has been changed and any View reflecting
the data set should refresh itself.*/
adapter.notifyDataSetChanged();
listView.setAdapter(adapter);
// OnCLickListener For List Items works the modify address aspect
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long
viewId) {
TextView idTextView = (TextView) view.findViewById(R.id.view_id);
TextView nameTextView = (TextView) view.findViewById(R.id.view_name);
TextView addressTextView = (TextView) view.findViewById(R.id.view_address);
id = idTextView.getText().toString();
name = nameTextView.getText().toString();
address = addressTextView.getText().toString();
Intent modify_intent = new Intent(getApplicationContext(), ModifyAddress.
class);
modify_intent.putExtra("name", name);
modify_intent.putExtra("address", address);
modify_intent.putExtra("id", id);
startActivity(modify_intent);
}
});
}
@Override
// loads the overflow menu on the bar
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == R.id.add_records) {
Intent add_mem = new Intent(this, AddAddress.class);
startActivity(add_mem);
}
return super.onOptionsItemSelected(item);
}
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////
package com.mycompany.dbase;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
public class ModifyAddress extends Activity implements OnClickListener {
private EditText nameText;
private Button updateBtn, deleteBtn;
private EditText addressText;
private long _id;
private DBManager dbManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setTitle("Modify Record");
setContentView(R.layout.modify_record);
dbManager = new DBManager(this);
dbManager.open();
nameText = (EditText) findViewById(R.id.name_edittext);
addressText = (EditText) findViewById(R.id.address_edittext);
updateBtn = (Button) findViewById(R.id.btn_update);
deleteBtn = (Button) findViewById(R.id.btn_delete);
Intent intent = getIntent();
String id = intent.getStringExtra("id");//id
String name = intent.getStringExtra("name");//("title");
String desc = intent.getStringExtra("address");//desc
_id = Long.parseLong(id);
nameText.setText(name);
addressText.setText(desc);
updateBtn.setOnClickListener(this);
deleteBtn.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_update:
String name = nameText.getText().toString();
String address = addressText.getText().toString();
dbManager.update(_id, name, address);
this.returnHome();
break;
case R.id.btn_delete:
dbManager.delete(_id);
this.returnHome();
break;
}
}
public void returnHome() {
Intent home_intent = new Intent(getApplicationContext(), MainActivity.class)
.setFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
startActivity(home_intent);
}
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////
package com.mycompany.dbase;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class SQLDatabaseHelper extends SQLiteOpenHelper {
// Table Name
public static final String TABLE_NAME = "ADDRESSES";
// Table columns
public static final String _ID = "_id";
public static final String NAME = "name";
public static final String ADDRESS = "address";
// Database Information
static final String DB_NAME = "MY_ADDRESSES.DB";//this name cannot match your TABLE name.
// database version
static final int DB_VERSION = 1;
// Creating table query
private static final String CREATE_TABLE = "create table " + TABLE_NAME + "(" + _ID
+ " INTEGER PRIMARY KEY AUTOINCREMENT, " + NAME + " TEXT NOT NULL, " + ADDRESS + "
TEXT);";
//Context (Activity), name of the database,("ADDRESS.DB")
// an optional cursor factory(null, and an integer
// representing your version of your database schema (1).
public SQLDatabaseHelper(Context context) {
super(context, DB_NAME, null, DB_VERSION);
}
@Override
//Called when the database is created for the first time.
public void onCreate(SQLiteDatabase db) {
db.execSQL(CREATE_TABLE);
}
@Override
// Called when the database needs to be upgraded.
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
/////////////////////////////////////////////////manifest.xmi////////////////////////////////////////////////////////
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android "
package="com.mycompany.dbase">
<!--ADDED BEGIN-->
<!-- <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>-->
<!-- ADDED END-->
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<!--ADDED BEGIN-->
<activity android:theme="@android:style/Theme.DeviceDefault.Light.Dialog"
android:name=".ModifyAddress"
android:label="@string/app_name" />
<activity android:theme="@android:style/Theme.DeviceDefault.Light.Dialog"
android:name=".AddAddress"
android:label="@string/app_name" />
<!-- ADDED END-->
</application>
</manifest>
/////////////////////////////////////////////////activity_main.xml////////////////////////////////////////////////////////
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android "
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="@drawable/bkg">
<ListView
android:id="@+id/list_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:dividerHeight="1dp"
android:padding="10dp"
android:background="#FFF">
</ListView>
<TextView
android:id="@+id/empty"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="@string/empty_list_text" />
</RelativeLayout>
//////////////////////////////////////////////////////add_record.xml///////////////////////////////////////////////////
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android "
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="20dp" >
<EditText
android:id="@+id/add_name_edittext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:hint="@string/enter_title" >
<requestFocus />
</EditText>
<EditText
android:id="@+id/add_address_edittext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:hint="@string/enter_desc"
android:inputType="textMultiLine"
android:minLines="5" >
</EditText>
<Button
android:id="@+id/add_add_record"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="@string/add_record" />
</LinearLayout>
/////////////////////////////////////////////////////////modify_record.xml////////////////////////////////////////////////
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android "
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="10dp" >
<EditText
android:id="@+id/name_edittext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:ems="10"
android:hint="@string/enter_title" />
<EditText
android:id="@+id/address_edittext"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:hint="@string/enter_desc"
android:inputType="textMultiLine"
android:minLines="5" >
</EditText>
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:weightSum="2"
android:gravity="center_horizontal"
android:orientation="horizontal" >
<Button
android:id="@+id/btn_update"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="@string/btn_update" />
<Button
android:id="@+id/btn_delete"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="@string/btn_delete" />
</LinearLayout>
</LinearLayout>
//////////////////////////////////////////////////////////view_record.xml///////////////////////////////////////////////
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android "
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical" >
<TextView
android:id="@+id/view_id"
android:layout_width="25dp"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginRight="6dp"
android:padding="3dp"
android:visibility="visible" />
<TextView
android:id="@+id/view_name"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_toRightOf="@id/view_id"
android:maxLines="1"
android:padding="3dp"
android:textSize="17sp"
android:textStyle="bold" />
<TextView
android:id="@+id/view_address"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_below="@id/view_name"
android:layout_marginLeft="10dp"
android:layout_toRightOf="@id/view_id"
android:ellipsize="end"
android:maxLines="2"
android:padding="3dp"
android:visibility="visible" />
</RelativeLayout>
//////////////////////////////////////////////////////menu.xml///////////////////////////////////////////////////
<menu xmlns:android="http://schemas.android.com/apk/res/android "
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context="com.example.sqlitesample.MainActivity" >
<item
android:id="@+id/add_records"
android:icon="@android:drawable/ic_menu_add"
android:orderInCategory="100"
android:title="@string/add_record"
app:showAsAction="always"/>
</menu>
/////////////////////////////////////////////////////////strings.xml////////////////////////////////////////////////
<resources>
<string name="app_name">SQLite Demo</string>
<string name="enter_name">Enter Name</string>
<string name="add_record">Add Record</string>
<string name="btn_update">Update</string>
<string name="btn_delete">Delete</string>
<string name="empty_list_text">No records found</string>
<string name="enter_desc">Address</string>
<string name="enter_title">Name</string>
</resources>