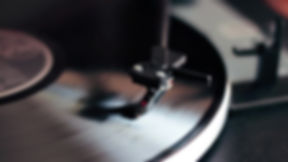
HLessJon Tutorials
Here is the link to the files I created for this project. There are two links to two differant cloud storage locations.
Dropbox:
https://www.dropbox.com/sh/jpqrzdsqsl0pyhv/AAADWGGTWpuRp_n9wJ__PB6oa?dl=0
Google Drive:
https://drive.google.com/folderview?id=0B7JGeHzm78HxN2FzazJRV2w1SE0&usp=sharing
package com.mycompany.musicalbuttonsone;
import android.content.Intent;
import android.content.SharedPreferences;
import android.media.MediaPlayer;
import android.os.Handler;
import android.preference.PreferenceManager;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.Toolbar;
import android.view.MotionEvent;
import android.view.View;
import android.widget.ImageView;
import android.view.Menu;
import android.view.MenuItem;
public class MainActivity extends AppCompatActivity {
//create all the variables
ImageView imageButtonLarge;
ImageView imageButtonMedium;
ImageView imageButtonSmall;
MediaPlayer soundLarge;
MediaPlayer soundMedium;
MediaPlayer soundSmall;
ImageView img1;
ImageView img2;
ImageView img3;
int buttonBig;
int buttonMed;
int buttonSml;
int buttonBig2;
int buttonMed2;
int buttonSml2;
/* int buttonBig = R.drawable.button_blue_a;
int buttonMed = R.drawable.button_red_a;
int buttonSml = R.drawable.button_yellow_a;
int buttonBig2 = R.drawable.button_blue_b;
int buttonMed2 = R.drawable.button_red_b;
int buttonSml2 = R.drawable.button_yellow_b;*/
int soundBig;
int soundMed;
int soundSml;
/* int soundBig = R.raw.low_piano;
int soundMed = R.raw.middle_piano;
int soundSml = R.raw.high_piano;*/
//part 2
String shapeChoice;
String instrumentChoice;
SharedPreferences prefs;
Toolbar mToolbar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
///part 2 Adding toolbar
mToolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(mToolbar);
getSupportActionBar().setDisplayShowHomeEnabled(true);
/////////
///part 2 ends
addListenerOnButton1();
addListenerOnButton2();
addListenerOnButton3();
refresher();
/*img1.setImageResource(buttonBig);
img2.setImageResource(buttonMed);
img3.setImageResource(buttonSml);*/
}
//part 2 Begins again
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
Intent i = new Intent(this, SettingsActivity.class);
// Start the refresh background task.
// This method calls setRefreshing(false) when it's finished.
startActivity(i);
/* Toast.makeText(getApplicationContext(), instrumentChoice +" shape", Toast.LENGTH_SHORT).show();*/
return true;
}
return super.onOptionsItemSelected(item);
}
/////////
@Override
protected void onResume() {
super.onResume();
refresher();
musical();
}
public void addListenerOnButton1() {
//////////////////PART2
imageButtonLarge = (ImageView) findViewById(R.id.imageView1);
// ImageView imageButtonLarge;
img1 = (ImageView) findViewById(R.id.imageView1);
// ImageView img1; already img1.setImageResource(buttonBig);
musical(); //attach sound to soundLarge
//soundBig assigned above
imageButtonLarge.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
soundLarge.start();//play sound
//TEST TOAST
/* Toast.makeText(getApplicationContext(),
instrumentChoice +" shape", Toast.LENGTH_SHORT).show();
*/
/* Toast.makeText(MainActivity.this,
"Large Clicked!",
Toast.LENGTH_SHORT).show();*/
img1.setImageResource(buttonBig2);
///load up the second button to indicate
//it has been pressed then use the handler
//to pause and return to original color
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
public void run() {
img1.setImageResource(buttonBig);
}
}, 200);
return false;
}
}); }
public void addListenerOnButton2() {
imageButtonMedium = (ImageView) findViewById(R.id.imageView2);
img2 = (ImageView) findViewById(R.id.imageView2);
musical(); //attach sound to soundMedium
imageButtonMedium.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
soundMedium.start();//play sound
/* Toast.makeText(MainActivity.this,
"Meduim Clicked!",
Toast.LENGTH_SHORT).show();*/
///load up the second button to indicate
//it has been pressed then use the handler
//to pause and return to original color
img2.setImageResource(buttonMed2);
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
public void run() {
img2.setImageResource(buttonMed);
}
}, 200);
return false;
}
}); }
public void addListenerOnButton3() {
imageButtonSmall = (ImageView) findViewById(R.id.imageView3);
img3 = (ImageView) findViewById(R.id.imageView3);
musical(); //attach sound to soundLarge
imageButtonSmall.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
soundSmall.start();//play sound
/* Toast.makeText(MainActivity.this,
"Small Clicked!",
Toast.LENGTH_SHORT).show();*/
///load up the second button to indicate
//it has been pressed then use the handler
//to pause and return to original color
img3.setImageResource(buttonSml2);
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
public void run() {
img3.setImageResource(buttonSml);
}
}, 200);
return false;
}
}); }
public void musical(){
prefs = PreferenceManager.getDefaultSharedPreferences(this);
instrumentChoice = prefs.getString("instrumentType", "1");
switch (instrumentChoice){
case "1":
soundLarge = MediaPlayer.create(this,R.raw.low_piano);
soundMedium = MediaPlayer.create(this,R.raw.middle_piano);
soundSmall = MediaPlayer.create(this, R.raw.high_piano);
break;
case "2":
soundLarge = MediaPlayer.create(this,R.raw.low_clarinet);
soundMedium = MediaPlayer.create(this,R.raw.middle_clarinet);
soundSmall = MediaPlayer.create(this, R.raw.high_clarinet);
break;
}
}
public void refresher() {
prefs = PreferenceManager.getDefaultSharedPreferences(this);
shapeChoice = prefs.getString("shapeType", "1");
switch (shapeChoice){
case "1": buttonBig = R.drawable.button_blue_a;
buttonMed = R.drawable.button_red_a;
buttonSml = R.drawable.button_yellow_a;
buttonBig2 = R.drawable.button_blue_b;
buttonMed2 = R.drawable.button_red_b;
buttonSml2 = R.drawable.button_yellow_b;
break;
case "2":
buttonBig = R.drawable.square_blue_a;
buttonMed = R.drawable.square_red_a;
buttonSml = R.drawable.square_yellow_a;
buttonBig2 = R.drawable.square_blue_b;
buttonMed2 = R.drawable.square_red_b;
buttonSml2 = R.drawable.square_yellow_b;
break;
case "3":
buttonBig = R.drawable.star_blue_a;
buttonMed = R.drawable.star_red_a;
buttonSml = R.drawable.star_yellow_a;
buttonBig2 = R.drawable.star_blue_b;
buttonMed2 = R.drawable.star_red_b;
buttonSml2 = R.drawable.star_yellow_b;
break;
}
img1.setImageResource(buttonBig);
img2.setImageResource(buttonMed);
img3.setImageResource(buttonSml);
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/main_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.mycompany.musicalbuttonsone.MainActivity"
android:background="#ffffff">
////////part 2
<include
android:id="@+id/toolbar"
layout="@layout/toolbar" />
///////part2
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView1"
android:layout_below="@+id/toolbar"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView2"
android:layout_below="@+id/imageView1"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp"
/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView3"
android:layout_below="@+id/imageView2"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp"
/>
</RelativeLayout>
package com.mycompany.musicalbuttonsone;
import android.os.Bundle;
import android.preference.PreferenceActivity;
import android.preference.PreferenceFragment;
public class SettingsActivity extends PreferenceActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
getFragmentManager().beginTransaction().replace(android.R.id.content, new MyPreferenceFragment()).commit();
}
public static class MyPreferenceFragment extends PreferenceFragment//the fragment
{
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
// Load the preferences from an XML resource
addPreferencesFromResource(R.xml.pref_headers);
}
}}
<?xml version="1.0" encoding="utf-8"?>
<android.support.v7.widget.Toolbar xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:local="http://schemas.android.com/apk/res-auto"
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:elevation="4dp"
android:minHeight="?attr/actionBarSize"
android:background="?attr/colorPrimary"/>
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/action_settings"
android:orderInCategory="1"
app:showAsAction="never"
android:title="@string/action_settings"/>
</menu>
1)
<resources>
<string-array name="listArray">
<item>Circles</item>
<item>Squares</item>
<item>Stars</item>
</string-array>
<string-array name="listValues">
<item>1</item>
<item>2</item>
<item>3</item>
</string-array>
</resources>
2)
<resources>
<string-array name="listArray2">
<item>Paino</item>
<item>Clarinet</item>
</string-array>
<string-array name="listValues2">
<item>1</item>
<item>2</item>
</string-array>
</resources>
colors
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#3F51B5</color>
<color name="colorPrimaryDark">#303F9F</color>
<color name="colorAccent">#FF4081</color>
</resources>
strings
<resources>
<string name="app_name">Musical Buttons One</string>
///part 2
<string name="action_settings">Settings</string>
//this is in the manifest and needs to stay here.
<string name="title_activity_settings">Settings</string>
</resources>
styles
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
</resources>
<?xml version="1.0" encoding="utf-8"?>
<PreferenceScreen xmlns:android="http://schemas.android.com/apk/res/android">
<ListPreference
android:title="Shape Type"
android:id="@+id/listPreferance1"
android:summary="Select the shape of your buttons"
android:key="shapeType"
android:entries="@array/listArray"
android:entryValues="@array/listValues" />
<ListPreference
android:title="Instrument Type"
android:id="@+id/listPreferance2"
android:summary="Select and instrument"
android:key="instrumentType"
android:entries="@array/listArray2"
android:entryValues="@array/listValues2" />
</PreferenceScreen>