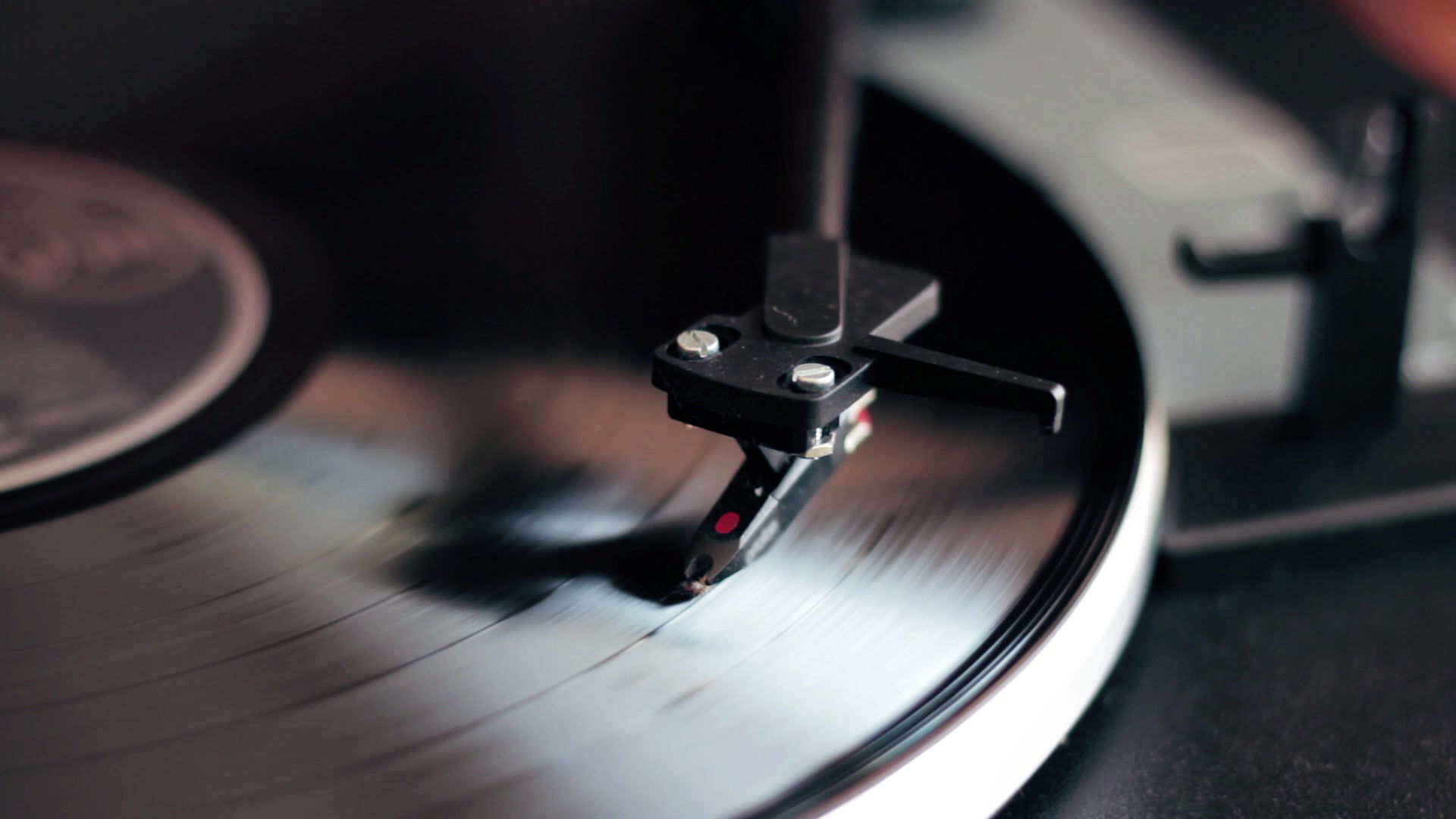
HLessJon Tutorials
Here is the link to the files I created for this project. There are two links to two differant cloud storage locations.
Dropbox:
https://www.dropbox.com/sh/jpqrzdsqsl0pyhv/AAADWGGTWpuRp_n9wJ__PB6oa?dl=0
Google Drive:
https://drive.google.com/folderview?id=0B7JGeHzm78HxN2FzazJRV2w1SE0&usp=sharing
package com.mycompany.musicalbuttonsone;
import android.media.MediaPlayer;
import android.os.Handler;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.MotionEvent;
import android.view.View;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
//create all the variables
ImageView imageButtonLarge;
ImageView imageButtonMedium;
ImageView imageButtonSmall;
MediaPlayer soundLarge;
MediaPlayer soundMedium;
MediaPlayer soundSmall;
ImageView img1;
ImageView img2;
ImageView img3;
int buttonBig = R.drawable.button_blue_a;
int buttonMed = R.drawable.button_red_a;
int buttonSml = R.drawable.button_yellow_a;
int buttonBig2 = R.drawable.button_blue_b;
int buttonMed2 = R.drawable.button_red_b;
int buttonSml2 = R.drawable.button_yellow_b;
int soundBig = R.raw.low_piano;
int soundMed = R.raw.middle_piano;
int soundSml = R.raw.high_piano;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
addListenerOnButton1();
addListenerOnButton2();
addListenerOnButton3();
img1.setImageResource(buttonBig);
img2.setImageResource(buttonMed);
img3.setImageResource(buttonSml);
}
public void addListenerOnButton1() {
imageButtonLarge = (ImageView) findViewById(R.id.imageView1);
// ImageView imageButtonLarge;
img1 = (ImageView) findViewById(R.id.imageView1);
// ImageView img1; already img1.setImageResource(buttonBig);
soundLarge = MediaPlayer.create(this,soundBig);//attach sound to soundLarge
//soundBig assigned above
imageButtonLarge.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
soundLarge.start();//play sound
Toast.makeText(MainActivity.this,
"Large Clicked!",
Toast.LENGTH_SHORT).show();
img1.setImageResource(buttonBig2);
///load up the second button to indicate
//it has been pressed then use the handler
//to pause and return to original color
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
public void run() {
img1.setImageResource(buttonBig);
}
}, 200);
return false;
}
}); }
public void addListenerOnButton2() {
imageButtonMedium = (ImageView) findViewById(R.id.imageView2);
img2 = (ImageView) findViewById(R.id.imageView2);
soundMedium = MediaPlayer.create(this,soundMed);//attach sound to soundMedium
imageButtonMedium.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
soundMedium.start();//play sound
Toast.makeText(MainActivity.this,
"Meduim Clicked!",
Toast.LENGTH_SHORT).show();
///load up the second button to indicate
//it has been pressed then use the handler
//to pause and return to original color
img2.setImageResource(buttonMed2);
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
public void run() {
img2.setImageResource(buttonMed);
}
}, 200); return false;
}
}); }
public void addListenerOnButton3() {
imageButtonSmall = (ImageView) findViewById(R.id.imageView3);
img3 = (ImageView) findViewById(R.id.imageView3);
soundSmall = MediaPlayer.create(this,soundSml);//attach sound to soundLarge
imageButtonSmall.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View v, MotionEvent event) {
soundSmall.start();//play sound
Toast.makeText(MainActivity.this,
"Small Clicked!",
Toast.LENGTH_SHORT).show();
///load up the second button to indicate
//it has been pressed then use the handler
//to pause and return to original color
img3.setImageResource(buttonSml2);
Handler handler = new Handler();
handler.postDelayed(new Runnable() {
public void run() {
img3.setImageResource(buttonSml);
}
}, 200);
return false;
}
}); }
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.mycompany.musicalbuttonsone.MainActivity"
android:background="#ffffff">
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView1"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:src="@drawable/button_blue_a" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView2"
android:layout_below="@+id/imageView1"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp"
android:src="@drawable/button_red_a" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView3"
android:layout_below="@+id/imageView2"
android:layout_centerHorizontal="true"
android:layout_marginTop="15dp"
android:src="@drawable/button_yellow_a" />
</RelativeLayout>