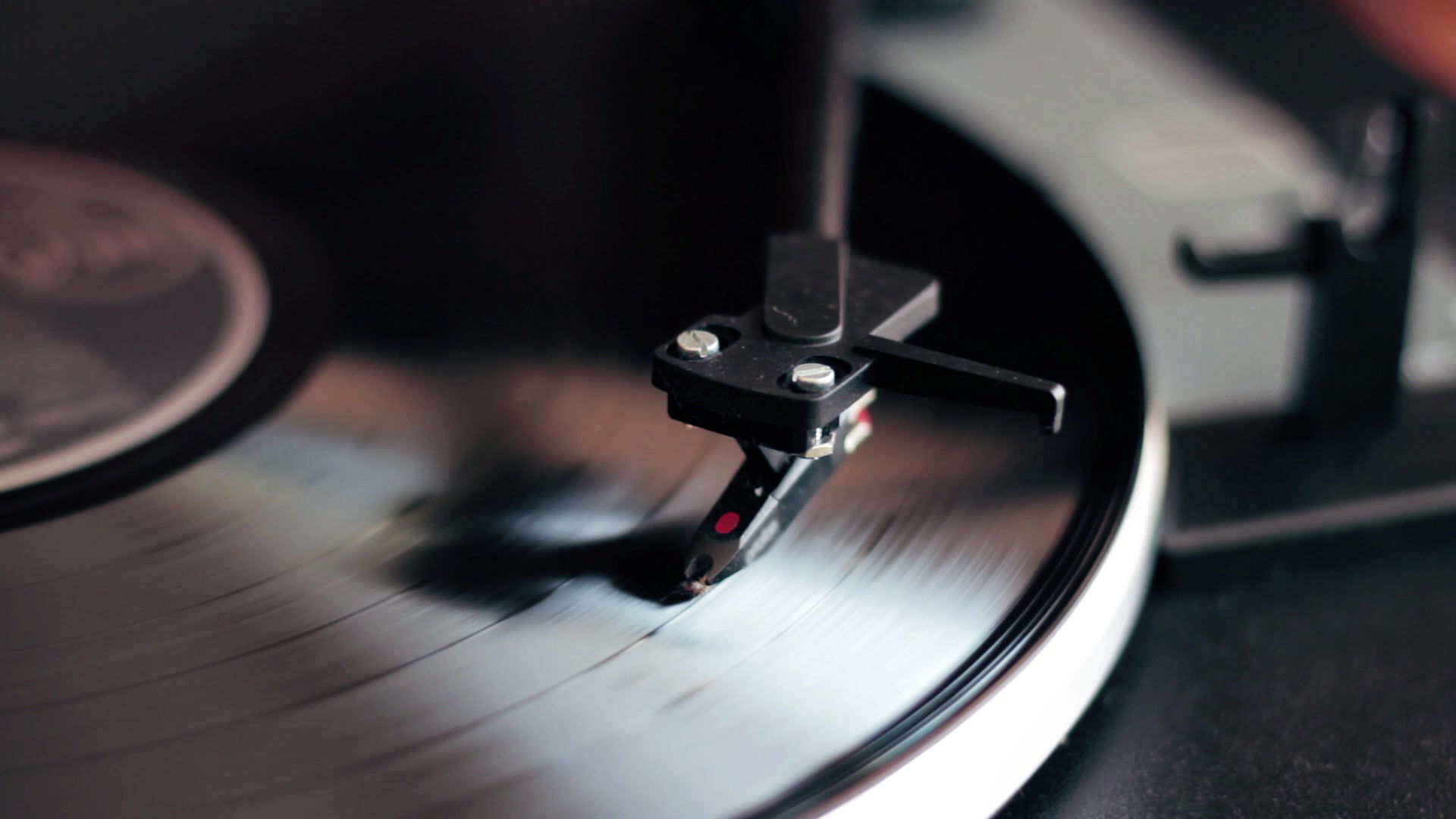
HLessJon Tutorials
package com.mycompany.twoactivities;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
//**20** because we need to use variables in more then on place declare them.
//**20** Define input fields
EditText editTextFirstName,editTextLastName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//**21** add get intent from ActivityTwo.java
//**21** use the already existing TextView to display our message
TextView display = (TextView) findViewById(R.id.primary);
//**21** used printHere as the Intent variable
Intent printHere = getIntent();
//**21** put in the string object we passed assign to a string variable
String name= printHere.getStringExtra("myReturnMessage");
//**21** display the text if there is something there.
//**21** if you dont use this if statement will still work but it just
//**21** shows an ugly null message "Primary report: null "
if(name != null){
display.setText("Primary report: " + name);}
editTextFirstName = (EditText) findViewById(R.id.editTextFirstName);
editTextLastName = (EditText) findViewById(R.id.editTextLastName);
//**20** buttonOne is only used here, so only declared here as a Button.
Button buttonOne = (Button) findViewById(R.id.button1);
// **20** assign buttonOne a listener
buttonOne.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
/***20** the manifest-file of our application and will display an
Activity if it finds one with the same class. ie ActivityTwo.class**20** */
Intent intent = new Intent(getApplicationContext(),ActivityTwo.class);
//**20** "fname" and "lname" must be used again in ActivityTwo so remember them.
//**20** Extract String objects with names fname and lname
intent.putExtra("fname", editTextFirstName.getText().toString());
intent.putExtra("lname", editTextLastName.getText().toString());
/***20** the putExtra method is used it adds a key-value pair to the object.
First parameter is key(name), the second - value.**20** */
//**20** we then send Intent using the startActivity method
startActivity(intent);
}
});
}
// **19** Replaced this code with the new code
/* **19** buttonOne.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v) {
// notice ActivityTwo is what we want to start
Intent intent = new Intent(getApplicationContext(),ActivityTwo.class);
// use startActivity() to start the new activity described by the intent
startActivity(intent);
}}**19** */
}
package com.mycompany.twoactivities;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
public class ActivityTwo extends AppCompatActivity {
TextView theView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_two);
//**20** Find TextView first
theView = (TextView) findViewById(R.id.theView);
Intent intent = getIntent();
//**20** receive Intent and extract String objects
//**20** "fname" and "lname"
String fName = intent.getStringExtra("fname");
String lName = intent.getStringExtra("lname");
theView.setText("Your name is: " + fName + " " + lName);
}
public void myClick(View v)
{
//**21** add EditText
EditText nameEdit = (EditText) findViewById(R.id.editTextReturn);
//c**21** create string to send
String returnMessage = nameEdit.getText().toString();
//**20**
Intent intent = new Intent(this,MainActivity.class);
//**21** use putExtra to send as myReturnMessage
intent.putExtra("myReturnMessage", returnMessage);
//**20**
startActivity(intent);
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.mycompany.twoactivities.MainActivity">
<!--**19**-->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Primary"
android:id="@+id/primary" />
<!--**19**-->
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button"
android:id="@+id/button1"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
<!--**20**-->
<EditText
android:layout_width="200dp"
android:layout_height="wrap_content"
android:id="@+id/editTextFirstName"
android:layout_below="@+id/primary"
android:layout_centerHorizontal="true"
android:layout_marginTop="39dp"
android:hint="First Name" />
<!--**20**-->
<EditText
android:layout_width="200dp"
android:layout_height="wrap_content"
android:id="@+id/editTextLastName"
android:layout_below="@+id/editTextFirstName"
android:layout_centerHorizontal="true"
android:layout_marginTop="30dp"
android:hint="Last Name" />
</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.mycompany.twoactivities.MainActivity">
<!--**19**-->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Secondary"
android:id="@+id/second" />
<!--**19**-->
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button"
android:id="@+id/button2"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true"
android:onClick="myClick"/> <!--**20**-->
<!--**20**-->
<TextView
android:layout_width="300dp"
android:layout_height="wrap_content"
android:text="First and Last Name"
android:id="@+id/theView"
android:layout_below="@+id/second"
android:layout_centerHorizontal="true"
android:layout_marginTop="65dp" />
<!--**21**-->
<EditText
android:layout_width="200dp"
android:layout_height="wrap_content"
android:id="@+id/editTextReturn"
android:layout_below="@+id/theView"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginTop="31dp"
android:hint="Return Message" />
</RelativeLayout>