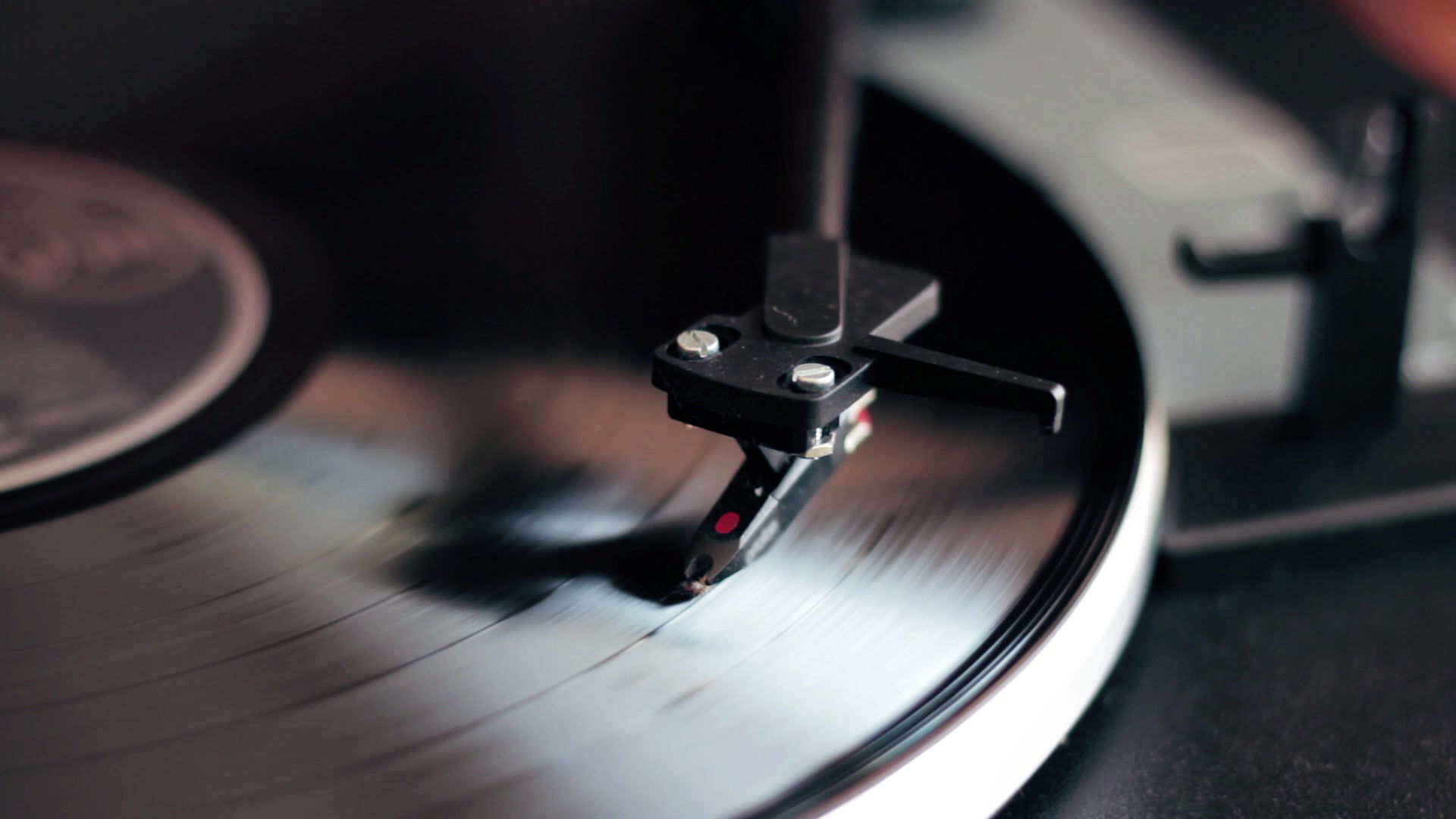
HLessJon Tutorials
package com.mycompany.arraysexample;
import android.graphics.Color;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.RelativeLayout;
import android.widget.Spinner;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//setContentView(R.layout.activity_main);
//CREATE layout and widget first
RelativeLayout relLay = new RelativeLayout(this);
//turn background to blue
relLay.setBackgroundColor(Color.BLUE);
Spinner newSpinner = new Spinner (this);
//adding new RelativeLayout
RelativeLayout.LayoutParams spannerParam =
new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.WRAP_CONTENT,
RelativeLayout.LayoutParams.WRAP_CONTENT
);
//add the rules
spannerParam.addRule(RelativeLayout.CENTER_VERTICAL);
spannerParam.addRule(RelativeLayout.CENTER_HORIZONTAL);
//add Spinner to the layout and include the new rules
relLay.addView(newSpinner,spannerParam);
//then add to the content view so you can see it
setContentView(relLay);
//Create a string array to hold the text to be displayed
String[] myStringArray={"Alpha","Bravo","Charlie"};
//Create the ArrayAdapter
ArrayAdapter<String> myAdapter=new
ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1,
myStringArray);
//Associate the ArrayAdapter with the ListView
Spinner myList= //Do not need to find byId we have the actual variable.
(Spinner) newSpinner;
myList.setAdapter((myAdapter));
//
}
}
package com.mycompany.arraysexample;
import android.graphics.Color;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.RelativeLayout;
import android.widget.Spinner;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//setContentView(R.layout.activity_main);
//CREATE layout and widget first
RelativeLayout relLay = new RelativeLayout(this);
//turn background to blue
relLay.setBackgroundColor(Color.BLUE);
Spinner newSpinner = new Spinner (this);
TextView newText = new TextView(this);
//text color
newText.setTextColor(Color.YELLOW);
//add the actual text
newText.setText("<Push Me!");
//assign IDs
newSpinner.setId(1);
newText.setId(2);
//adding new RelativeLayout
RelativeLayout.LayoutParams spannerParam =
new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.WRAP_CONTENT,
RelativeLayout.LayoutParams.WRAP_CONTENT
);
RelativeLayout.LayoutParams textViewParam =
new RelativeLayout.LayoutParams(
RelativeLayout.LayoutParams.WRAP_CONTENT,
RelativeLayout.LayoutParams.WRAP_CONTENT
);
//add the rules
spannerParam.addRule(RelativeLayout.CENTER_VERTICAL);
spannerParam.addRule(RelativeLayout.CENTER_HORIZONTAL);
textViewParam.addRule(RelativeLayout.CENTER_VERTICAL);
textViewParam.addRule(RelativeLayout.RIGHT_OF, newSpinner.getId());
textViewParam.setMargins(40,0,0,0);
//add Spinner to the layout and include the new rules
relLay.addView(newSpinner,spannerParam);
relLay.addView(newText,textViewParam);
//then add to the content view so you can see it
setContentView(relLay);
//Create a string array to hold the text to be displayed
String[] myStringArray={"Alpha","Bravo","Charlie"};
//Create the ArrayAdapter
ArrayAdapter<String> myAdapter=new
ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1,
myStringArray);
//Associate the ArrayAdapter with the ListView
Spinner myList= //Do not need to find byId we have the actual variable.
(Spinner) newSpinner;
myList.setAdapter((myAdapter));
//
}
}