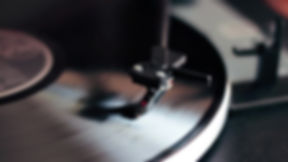
HLessJon Tutorials
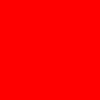

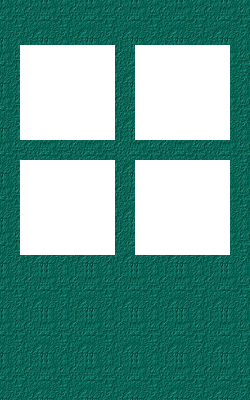
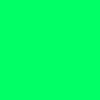
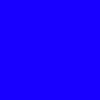
package com.mycompany.graphictest;
import android.app.Activity;
import android.view.View.OnClickListener;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends Activity implements OnClickListener {
private ImageView blue,green, red, yellow;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//
blue = (ImageView) findViewById(R.id.blue_sqr);
green = (ImageView) findViewById(R.id.green_sqr);
red = (ImageView) findViewById(R.id.red_sqr);
yellow = (ImageView) findViewById(R.id.yellow_sqr);
//set the listeners
blue.setOnClickListener(this);
//GREEN Listener
green.setOnClickListener(this);
//commented out because not going to use it this way.
/* red.setOnClickListener(this);
yellow.setOnClickListener(this);*/
red.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(getApplicationContext(), "Red", Toast.LENGTH_SHORT).show();
}
});
//YELLOW HERE!!
yellow.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v){
Toast.makeText(getApplicationContext(),"Yellow",Toast.LENGTH_SHORT).show();
}
});
}
@Override
public void onClick (View v)
{
switch (v.getId()) {
case R.id.blue_sqr:
Toast.makeText(getApplicationContext(), "Blue", Toast.LENGTH_SHORT).show();
break;
//GREEN
case R.id.green_sqr:
Toast.makeText(getApplicationContext(),"Green",Toast.LENGTH_SHORT).show();
}
}}
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_height="match_parent"
android:layout_width="match_parent"
android:padding="10dp">
<!--First layer -> Base layer-->
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="-110dp"
android:layout_marginLeft="-60dp"
android:id="@+id/blue_sqr"
android:src="@drawable/blue_sq" />
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_marginLeft="60dp"
android:id="@+id/red_sqr"
android:src="@drawable/red_sq" />
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_marginLeft="-60dp"
android:id="@+id/yellow_sqr"
android:src="@drawable/yellow_sq" />
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="-110dp"
android:layout_marginLeft="60dp"
android:id="@+id/green_sqr"
android:src="@drawable/green_sq" />
<!--Top layer-->
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/willMouth"
android:src="@drawable/four_frames_green_pat" />
</FrameLayout>
<?xml version="1.0" encoding="utf-8"?>
<!--Container of the three layers-->
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_height="match_parent"
android:layout_width="match_parent"
android:padding="10dp">
<!--First layer -> Base layer-->
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="-85dp"
android:layout_marginLeft="-45dp"
android:id="@+id/blue_sqr"
android:src="@drawable/blue_sq" />
<!--Second layer-->
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_marginLeft="45dp"
android:id="@+id/red_sqr"
android:src="@drawable/red_sq" />
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:layout_marginLeft="-45dp"
android:id="@+id/yellow_sqr"
android:src="@drawable/yellow_sq" />
<!--Second layer-->
<ImageView
android:layout_gravity="center"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="-85dp"
android:layout_marginLeft="50dp"
android:id="@+id/green_sqr"
android:src="@drawable/green_sq" />
<!--Top layer-->
<ImageView
android:layout_gravity="center"
android:layout_width="270dp"
android:layout_height="320dp"
android:id="@+id/frame"
android:src="@drawable/four_frames_green_pat" />
</FrameLayout>