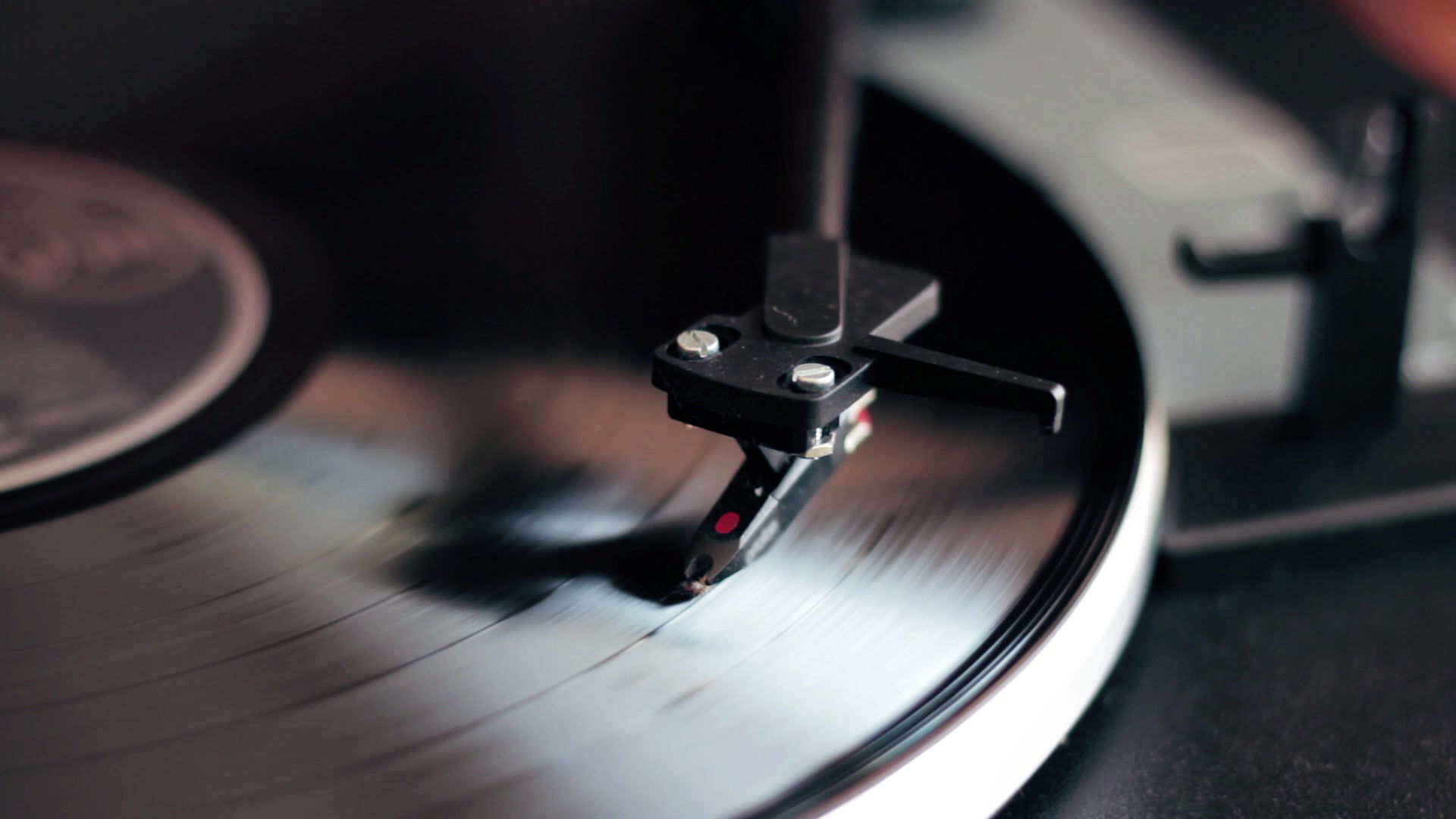
HLessJon Tutorials
package com.mycompany.multipanefragment;
import android.content.res.Configuration;
import android.os.Bundle;
import android.support.v4.app.FragmentActivity;
import android.view.View;
public class MyActivity extends FragmentActivity {
/*A fragment must always be embedded in an activity
and the fragment's lifecycle is directly affected by
the host activity's lifecycle.*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
// Hides the side panel when in portrait mode HIGHLIGHT THIS
// SECTION AND COMMENT IT OUT TO SEE WHAT HAPPENS
int screenOrientation = getResources().getConfiguration().orientation;
if (screenOrientation == Configuration.ORIENTATION_PORTRAIT) {
hideSidePane();
}
}
// Hides the side panel
private void hideSidePane() {
View sidePane = findViewById(R.id.side_panel);
if (sidePane.getVisibility() == View.VISIBLE) {
sidePane.setVisibility(View.GONE);
}
}
}
package com.mycompany.multipanefragment;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class ButtonPanelFragment extends Fragment {
@Override
/* The container parameter passed to onCreateView()
is the parent ViewGroup (from the activity's layout)
in which your fragment layout will be inserted. */
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return inflater.inflate(R.layout.button_panel, container, false);
/*
The inflate() method takes three arguments:
1) The resource ID of the layout you want to inflate.
2) The ViewGroup to be the parent of the inflated layout. Passing the container
is important in order for the system to apply layout parameters to the root view
of the inflated layout, specified by the parent view in which it's going.
3)A boolean indicating whether the inflated layout should be attached to the ViewGroup
(the second parameter) during inflation. (In this case, this is false because the system
is already inserting the inflated layout into the container—passing true would create
a redundant view group in the final layout.)
*/
}
}
package com.mycompany.multipanefragment;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
/* A fragment is like a modular section of an activity,
which has its own lifecycle, receives its own input events,
and which you can add or remove while the activity is running*/
//To create a fragment, extend the Fragment class
public class MainPanelFragment extends Fragment {
/*
LayoutInflator takes the xml layout and puts it in a "view
container" is the view the fragment should be attached to
savedInstanceState is passed if the fragment is being re-constructed from a saved state
inflate() is passed the layout xml to place, the optional view to attach to and
true or false on whether to attach to the optional view named container
*/
@Override
/* R.layout.main_panel is a reference to a layout resource named main_panel.xml
saved in the application resources*/
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.main_panel, container, false);
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/RelativeLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:baselineAligned="false"
android:animateLayoutChanges="true"
tools:context=".MyActivity" >
<fragment
android:id="@+id/main_panel"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
class="com.mycompany.multipanefragment.MainPanelFragment" /> <!--pointing at your fragment-->
<fragment
android:id="@+id/side_panel"
android:layout_width="155dp"
android:layout_height="match_parent"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
class="com.mycompany.multipanefragment.ButtonPanelFragment" /> <!--pointing at your fragment-->
</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/RelativeLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:baselineAligned="false"
android:animateLayoutChanges="true"
tools:context=".MyActivity" >
<!-- Declare the fragment inside the activity's layout file, you can specify layout
properties for the fragment as if it were a view.-->
<fragment
android:id="@+id/main_panel"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
class="com.mycompany.multipanefragment.MainPanelFragment" /> <!--pointing at your fragment -->
<!--each instance of a Fragment class must be associated with a parent FragmentActivity here .MainPanelFragment-->
<!--represent as or by an instance-->
<!-- When the system creates this activity layout, it <instantiates> each fragment specified in the layout
and calls the onCreateView() method for each one, to retrieve each fragment's layout. The system inserts
the View returned by the fragment directly in place of the <fragment> element.-->
<fragment
android:id="@+id/side_panel"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
class="com.mycompany.multipanefragment.ButtonPanelFragment" /> <!--pointing at your fragment-->
</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#44a8ff"
android:gravity="top|center"
android:orientation="vertical" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Main Panel"
android:textColor="@android:color/white"
android:textStyle="bold"
android:textSize="20sp"/>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#77ad39"
android:gravity="top|center"
android:orientation="vertical" >
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="Button Panel"
android:id="@+id/button"
android:layout_gravity="center_horizontal" />
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="New Button"
android:id="@+id/button2"
android:layout_gravity="center_horizontal" />
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="New Button"
android:id="@+id/button3"
android:layout_gravity="center_horizontal" />
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="New Button"
android:id="@+id/button4"
android:layout_gravity="center_horizontal" />
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="New Button"
android:id="@+id/button5"
android:layout_gravity="center_horizontal" />
</LinearLayout>