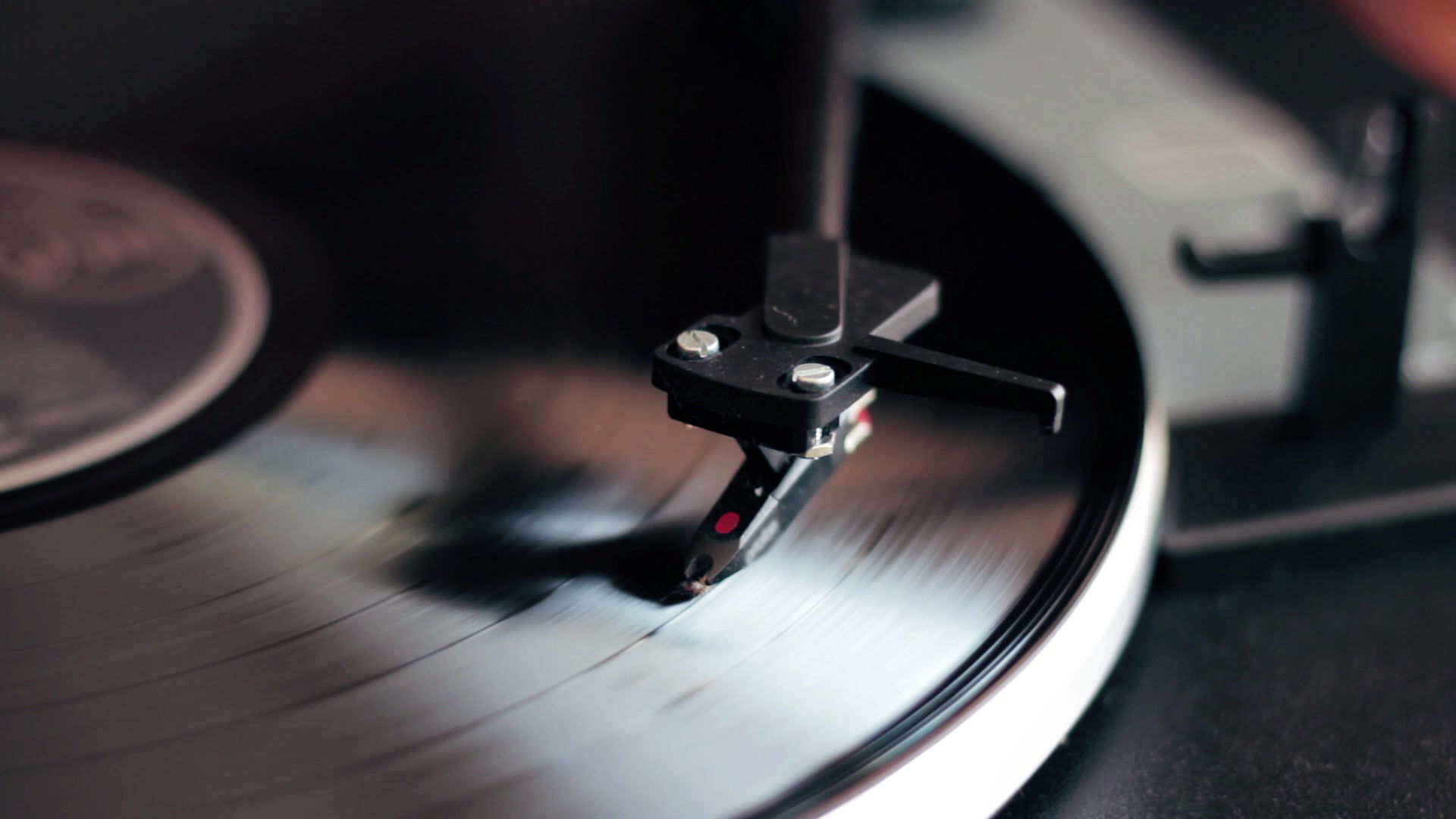
HLessJon Tutorials
package com.mycompany.reaction;
import android.graphics.Color;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.Spinner;
import android.widget.TextView;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
ArrayList<String> colorList = new ArrayList<String>();
Button myButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//there are a lot of things your going to need to import ALT+ENTER import class.
//create spinner in memory
final Spinner spinner = (Spinner) findViewById(R.id.spinner1);//name is important in xml
// this is your list that goes in the spinner
colorList.add("Choose");
colorList.add("Red");
colorList.add("Blue");
// Create the ArrayAdapter
ArrayAdapter<String> arrayAdapter = new ArrayAdapter<String>(MainActivity.this
,android.R.layout.simple_spinner_item,colorList);
// Set the Adapter
spinner.setAdapter(arrayAdapter);
// Set the ClickListener for Spinner
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
public void onItemSelected(AdapterView<?> adapterView,
View view, int i, long l) {
//make your choices
//this String.valueOf(spinner.getSelectedItem())turns
// whatever your spinner choice is into a string you can use
// assigned it to a variable to make it faster to work with
String choice = String.valueOf(spinner.getSelectedItem());
if (choice == "Red") {//do stuff here,
RelativeLayout backChange = (RelativeLayout) findViewById(R.id.re_bck);
//need to name your RelativeLayout re_bck for this to work
backChange.setBackgroundColor(Color.RED);
} else if (choice == "Blue") {//do stuff here,
RelativeLayout backChange = (RelativeLayout) findViewById(R.id.re_bck);
//need to name your RelativeLayout re_bck for this to work
backChange.setBackgroundColor(Color.BLUE);
}// this is affect the default color because Blue or Red is not chosen.
else {
choice = "White";
RelativeLayout backChange = (RelativeLayout) findViewById(R.id.re_bck);
backChange.setBackgroundColor(Color.WHITE);
}
///
myButton = (Button)findViewById(R.id.button1);
final String finalChoice = choice;
myButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(finalChoice == "Blue"){
TextView myText = (TextView)findViewById(R.id.textView1);
myText.setText("Blue");}
else if (finalChoice == "Red"){
TextView myText = (TextView)findViewById(R.id.textView1);
myText.setText("Red");}
else{
TextView myText = (TextView)findViewById(R.id.textView1);
myText.setText("White");}
}
});
///
}
// If no option selected
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
}
});
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.mycompany.reaction.MainActivity"
android:id="@+id/re_bck">
<Spinner
android:id="@+id/spinner1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="left"
android:layout_alignParentTop="true"
android:layout_marginTop="184dp" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me"
android:id="@+id/button1"
android:layout_alignTop="@+id/spinner1"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="What Color?"
android:id="@+id/textView1"
android:layout_below="@+id/button1"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:layout_alignLeft="@+id/button1"
android:layout_alignStart="@+id/button1"
android:background="#ffffff" />
</RelativeLayout>