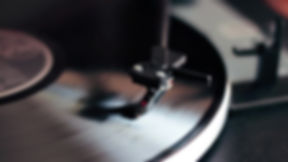
HLessJon Tutorials
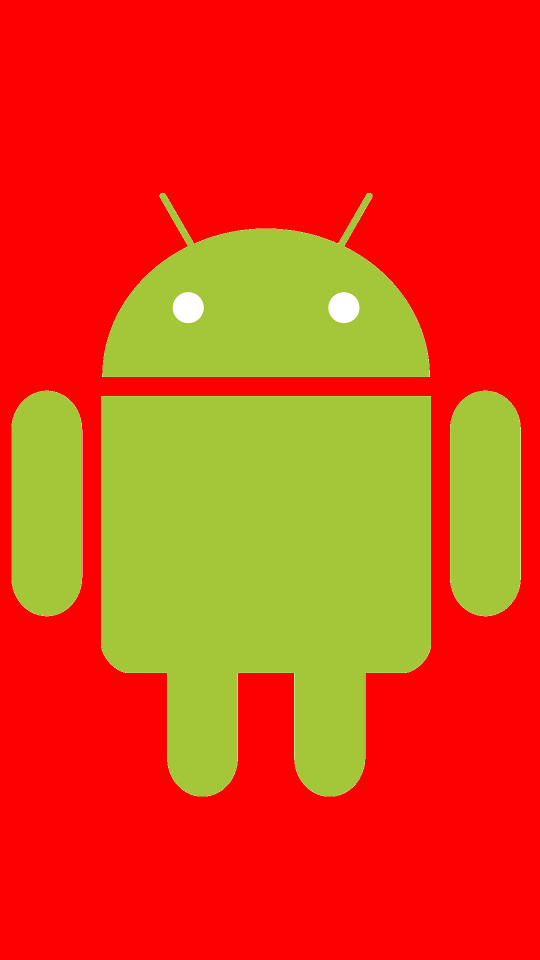
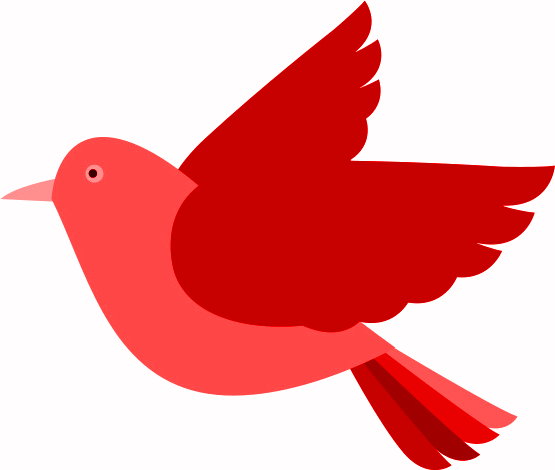
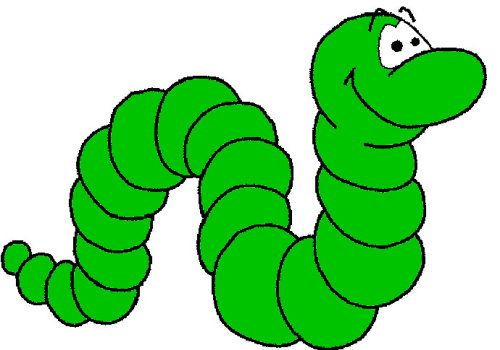
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#ff0202"
android:gravity="top|center"
android:orientation="vertical" >
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:background="#ff0202"
android:src="@drawable/robbit1"/>
</LinearLayout>
package com.mycompany.multipanefragment;
import android.app.Activity;
import android.app.Fragment;
import android.app.FragmentTransaction;
import android.os.Bundle;
public class MyActivity extends Activity {
Fragment frag;
FragmentTransaction fragTran;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_my);
frag = new MainPanelFragment();
fragTran = getFragmentManager().beginTransaction().add(R.id.primary_panel,frag);
fragTran.commit();
frag = new ButtonPanelFragment();
fragTran = getFragmentManager().beginTransaction().add(R.id.side_panel,frag);
fragTran.commit();
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/RelativeLayout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:baselineAligned="false"
android:animateLayoutChanges="true"
tools:context=".MyActivity" >
<!-- Declare the fragment inside the activity's layout file, you can specify layout
class="com.mycompany.multipanefragment.MainPanelFragment"
properties for the fragment as if it were a view.-->
<!--<fragment ; originally a <fragment> was used but replace that with a named layout-->
<FrameLayout
android:id="@+id/primary_panel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true"
android:layout_toRightOf="@+id/side_panel"
android:layout_toEndOf="@+id/side_panel" />
<!--used to contain android:name="com.mycompany.multipanefragment.MainPanelFragment"-->
<!--<fragment ; originally a <fragment> was used but replace that with a named layout-->
<FrameLayout
android:id="@+id/side_panel"
android:layout_width="130dp"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_alignParentBottom="true" />
<!--orignially used class="com.mycompany.multipanefragment.ButtonPanelFragment"-->
</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_height="match_parent"
android:layout_width="match_parent"
android:background="#77ad39"
android:id="@+id/Bttn_Layout"
android:orientation="vertical" >
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="Robot"
android:id="@+id/button1" />
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="Wurm"
android:id="@+id/button2"
android:layout_below="@+id/button1"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<Button
android:layout_width="125dp"
android:layout_height="wrap_content"
android:text="Bird"
android:id="@+id/button3"
android:layout_below="@+id/button2"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
</RelativeLayout>
package com.mycompany.multipanefragment;
import android.os.Bundle;
import android.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class MainPanelFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return inflater.inflate(R.layout.main_panel, container, false);
}
}
package com.mycompany.multipanefragment;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
public class ButtonPanelFragment extends Fragment {
Fragment frag;
FragmentTransaction fragTran;
/* public ButtonPanelFragment() {
}*/
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.button_panel, container, false);
/* frag = new MainPanelFragment();
fragTran = getFragmentManager().beginTransaction().replace(R.id.primary_panel, frag);
fragTran.commit();*/
Button button1 = (Button) view.findViewById(R.id.button1);
Button button2 = (Button) view.findViewById(R.id.button2);
Button button3 = (Button) view.findViewById(R.id.button3);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
frag = new MainPanelFragment();
fragTran = getFragmentManager().beginTransaction().replace(R.id.primary_panel, frag);
fragTran.commit();
}
});
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
frag = new MainPanelFragment2();
fragTran = getFragmentManager().beginTransaction().replace(R.id.primary_panel, frag);
fragTran.commit();
}
});
button3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
frag = new MainPanelFragment3();
fragTran = getFragmentManager().beginTransaction().replace(R.id.primary_panel, frag);
fragTran.commit();
}
});
return view;
}
}