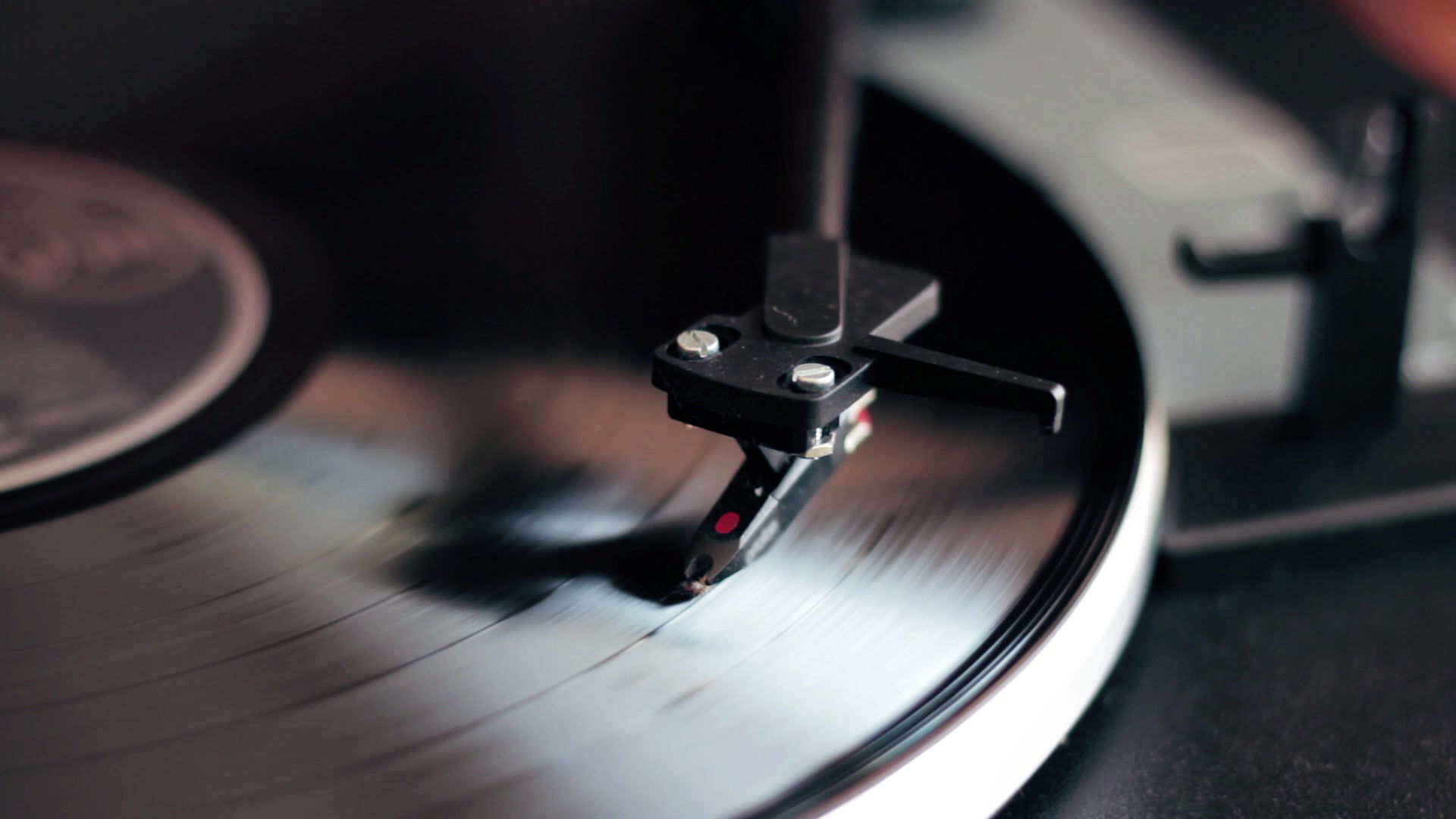
HLessJon Tutorials
package com.mycompany.fragmentdemo;
import android.app.Activity;
import android.support.v4.app.Fragment;
import android.app.FragmentManager;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends Activity {
Button clickButton;
boolean status = false;//set our boolean up to load FragmentOne
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
clickButton = (Button)findViewById(R.id.btn1);
clickButton.setText("Press to Load First Fragment");
clickButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
/* ///////////////////////////////// could put these outside
of the if statements and it would work as well
FragmentManager fragmentManager = getFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
///////////////////////////////// */
//if the value of the boolean status is true
if (status == false)//so first button click have to load the first fragment
{//have to create an object of the first fragment to begin.
FragmentOne frag1 = new FragmentOne();//step 1
//when you load
FragmentManager fragmentManager = getFragmentManager(); //step3
//we are not using intent here so we skill step 2
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
//now load the first fragement into the relative layout
//waiting for it on activity_main.xml
fragmentTransaction.add(R.id.target_container,frag1);//arguments are the container for the frag
//if you get an error here, one problem might be in your FragmentOne
//you are importing import android.support.v4.app.Fragment;
//you should try import android.app.Fragment;
//this error is often caused by loading the wrong libraries.
//How do you know? Read the library documents
// or just get to know what causes what errors and fix it.
fragmentTransaction.commit();
//now change the text on the button
clickButton.setText("Load 2nd Frag");
status = true;
}
else{
FragmentTwo frag2 = new FragmentTwo();//step 1
//we are not using intent here so we skill step 2
FragmentManager fragmentManager = getFragmentManager(); //step3
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();//step 4
fragmentTransaction.add(R.id.target_container, frag2);//step 5
fragmentTransaction.commit();//step 6
clickButton.setText("Load 1st Frag");
status = false;
}
}
});
}
}
package com.mycompany.fragmentdemo;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
/**
* A simple {@link Fragment} subclass.
*/
public class FragmentOne extends Fragment {
public FragmentOne() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_one_layout, container, false);
}
}
package com.mycompany.fragmentdemo;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
/**
* A simple {@link Fragment} subclass.
*/
public class FragmentTwo extends Fragment {
public FragmentTwo() {
// Required empty public constructor
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_two_layout, container, false);
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:andriod="http://schemas.android.com/apk/res-auto"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:orientation="vertical">
<Button
android:layout_width="250dp"
android:layout_height="wrap_content"
android:text="Load First Fragment"
android:id="@+id/btn1"
android:layout_gravity="center" />
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="400dp"
android:layout_marginTop="10dp"
android:id="@+id/target_container"/>
</LinearLayout>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.mycompany.fragmentdemo.FragmentOne"
android:background="#ff0000">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="@string/hello_blank_fragment"
android:layout_gravity="center"
android:gravity="center"
android:textAppearance="?android:textAppearanceLarge"/>
</FrameLayout>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.mycompany.fragmentdemo.FragmentOne"
android:background="#62c186">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Welcome to two"
android:layout_gravity="center"
android:gravity="center"
android:textAppearance="?android:textAppearanceLarge"/>
</FrameLayout>