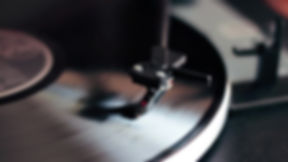
HLessJon Tutorials
package main.example.com.myapplication;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private CheckBox check1, check2, check3;
private Button button_select;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
addListnerOnButton ();
}
public void addListnerOnButton (){
check1=(CheckBox)findViewById(R.id.checkBox_red);
check2=(CheckBox)findViewById(R.id.checkBox2_blue);
check3=(CheckBox)findViewById(R.id.checkBox3_yellow);
button_select=(Button)findViewById(R.id.button);
button_select.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
StringBuffer result = new StringBuffer();
result.append("Red: ").append(check1.isChecked());
result.append("Blue: ").append(check2.isChecked());
result.append("Yellow: ").append(check3.isChecked());
Toast.makeText(MainActivity.this, result.toString(),
Toast.LENGTH_SHORT).show();
}
} );
}
}
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.main.myapplication.MainActivity">
<CheckBox
android:layout_width="400dp"
android:layout_height="wrap_content"
android:text="Red"
android:id="@+id/checkBox_red"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:checked="false"
android:background="#ff0000" />
<CheckBox
android:layout_width="400dp"
android:layout_height="wrap_content"
android:text="Blue"
android:id="@+id/checkBox2_blue"
android:layout_below="@+id/checkBox_red"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginTop="49dp"
android:checked="false"
android:background="#0011ff" />
<CheckBox
android:layout_width="400dp"
android:layout_height="wrap_content"
android:text="Yellow"
android:id="@+id/checkBox3_yellow"
android:checked="false"
android:background="#ffe600"
android:layout_above="@+id/button"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginBottom="24dp" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Select"
android:id="@+id/button"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
</RelativeLayout>