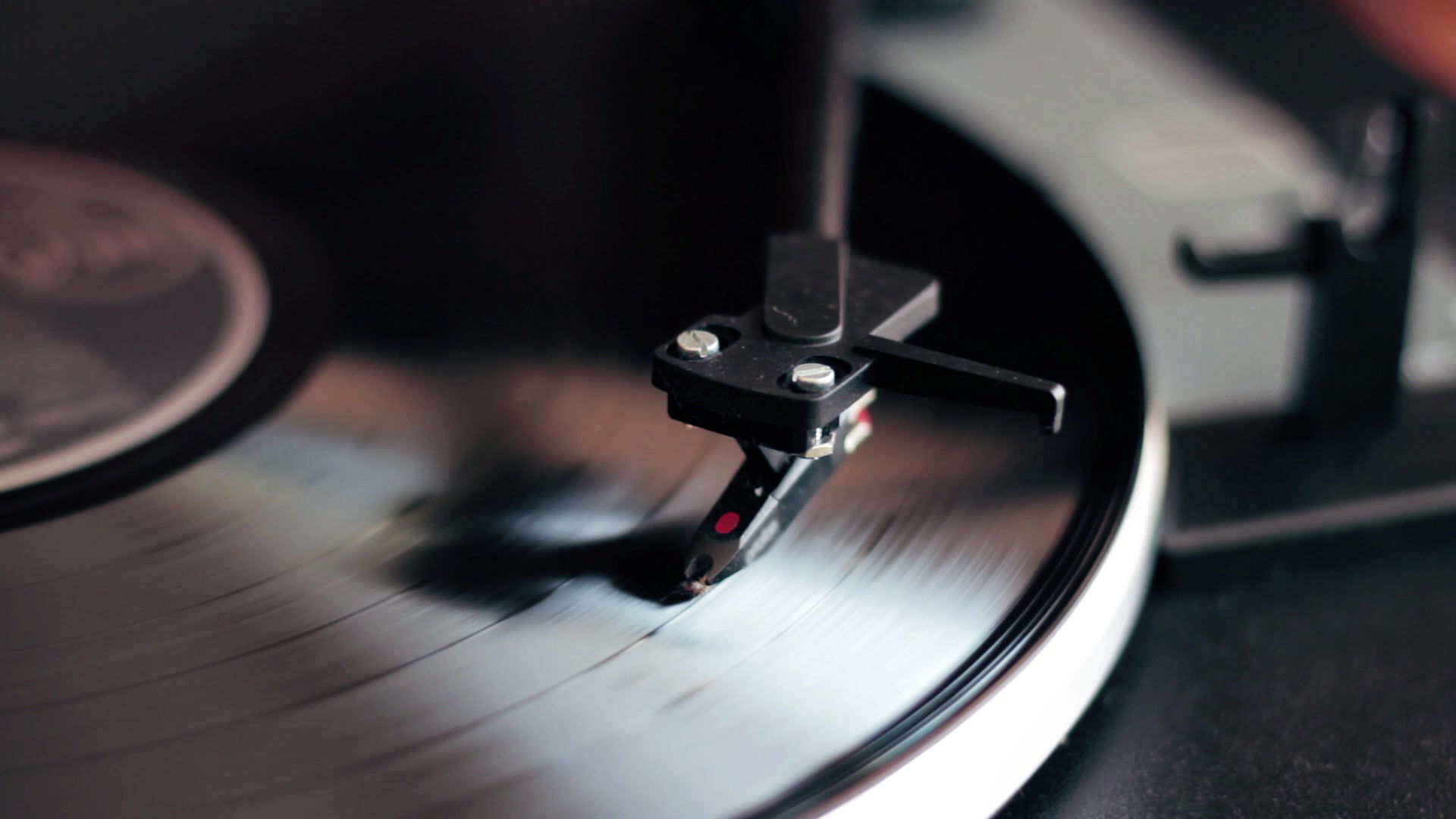
HLessJon Tutorials
package com.mycompany.aboutbundle;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.Button;
public class MainActivity extends Activity implements View.OnClickListener {
private final int SECONDARY_ACTIVITY_REQUEST_CODE = 0;
private EditText mEditText1;
private EditText mEditText2;
private Button mButton1;
//DONT FORGET: to add SecondaryActivity to the manifest file!!!!!
/**
* Called when the activity is first created.
*/
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mEditText1 = (EditText) findViewById(R.id.editText1);
mEditText2 = (EditText) findViewById(R.id.editText1b);
mButton1 = (Button) findViewById(R.id.button1);
mButton1.setOnClickListener(this);
}
public void onClick(View view) {
if (view == mButton1) {
//create a new intent and specify that the target is SecondaryActivity...
Intent intent = new Intent(getApplicationContext(), SecondaryActivity.class);
//load the intent with a key "myKey" and assign a value
//to be whatever has been entered into the textview
//Notice this only sends mEditText1 to SecondaryActivity.java inside intent using putExtra
//which is why the data sent from SecondaryActivity is seen in MainActivity but you are not
//sending info in mEditText2 back to SecondaryActivity and thus
// SecondaryActivity will always load txtSecondary2 empty
intent.putExtra("myKey", mEditText1.getText().toString());
//launch the secondary activity and send the intent along with it
//note that a request code is passed in as well so that when the
//secondary activity returns control to this activity,
//we can identify the source of the request...
startActivityForResult(intent, SECONDARY_ACTIVITY_REQUEST_CODE);
}
}
//we need a handler for when the secondary activity finishes it's work
//and returns control to this onActivity, also preserves initial data entry.
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent intent) {
super.onActivityResult(requestCode, resultCode, intent);
Bundle extras = intent.getExtras();
// get your data out of the bundle using the get methods:
//could also use
//mEditText2.setText(extras != null ? extras.getString("returnKey"): "nothing returned");
//Check if bundle contains key or not then handle that event
if(extras.containsKey("returnKey")){
mEditText2.setText(extras.getString("returnKey"));
}else{
mEditText2.setText("Nothing Returned");
}
}
}
package com.mycompany.aboutbundle;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class SecondaryActivity extends Activity{
private EditText mEditText2;
private EditText mEditText2b;//txtSecondary2 the bottom textview**
private Button mButton2;
private String mIntentString;
@Override
public void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
setContentView(R.layout.secondary_activity);
mEditText2 = (EditText)findViewById(R.id.txtSecondary);
mEditText2b = (EditText)findViewById(R.id.txtSecondary2);//txtSecondary2 the bottom textview**
mButton2 = (Button)findViewById(R.id.btnSecondary);
//event handlers for button
mButton2.setOnClickListener(new View.OnClickListener(){
public void onClick(View view){
mIntentString = mEditText2.getText().toString();
//create a new intent...
Intent intent = new Intent();
//add "returnKey" as a key and assign it the value
//in the textbox...
intent.putExtra("returnKey",mEditText2b.getText().toString());//txtSecondary2 the bottom textview**
//get ready to send the result back to the caller (MainActivity)
//and put our intent into it (RESULT_OK will tell the caller that
//we have successfully accomplished our task..
setResult(RESULT_OK,intent);
//close this Activity...
finish();
}
});
//check if savedInstance is not null and and if it is not then getString and give it myKey
mIntentString = savedInstanceState != null ? savedInstanceState.getString("myKey"):null;
//check to see if a Bundle is NULL or not
if(mIntentString == null){
//get the Bundle out of the Intent...
Bundle extras = getIntent().getExtras();
//Your app could crash if you try and get data out of the intent and there is no data there.
// Use your key to check if your data is in the bundle
// assign "nothing passed in" to mIntentString if empty
mIntentString = extras != null ? extras.getString("myKey") : "nothing passed in";
}
//set the textview to display mIntentString...
mEditText2.setText(mIntentString);
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.mycompany.aboutbundle">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".SecondaryActivity"/>
</application>
</manifest>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="MAIN ACTIVITY"/>
<EditText android:id="@+id/editText1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:hint="Send and Saves " />
<Button android:id="@+id/button1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Click To Launch Secondary Activity"/>
<EditText
android:id="@+id/editText1b"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:hint="Recieves But Does Not Send" />
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="SECONDARY ACTIVITY"/>
<EditText android:id="@+id/txtSecondary"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:hint="Info From First Act" />
<Button android:id="@+id/btnSecondary"
android:text="Click To Return to Main Activity"
android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/txtSecondary2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Info For First Act"
android:layout_gravity="center_horizontal" />
</LinearLayout>